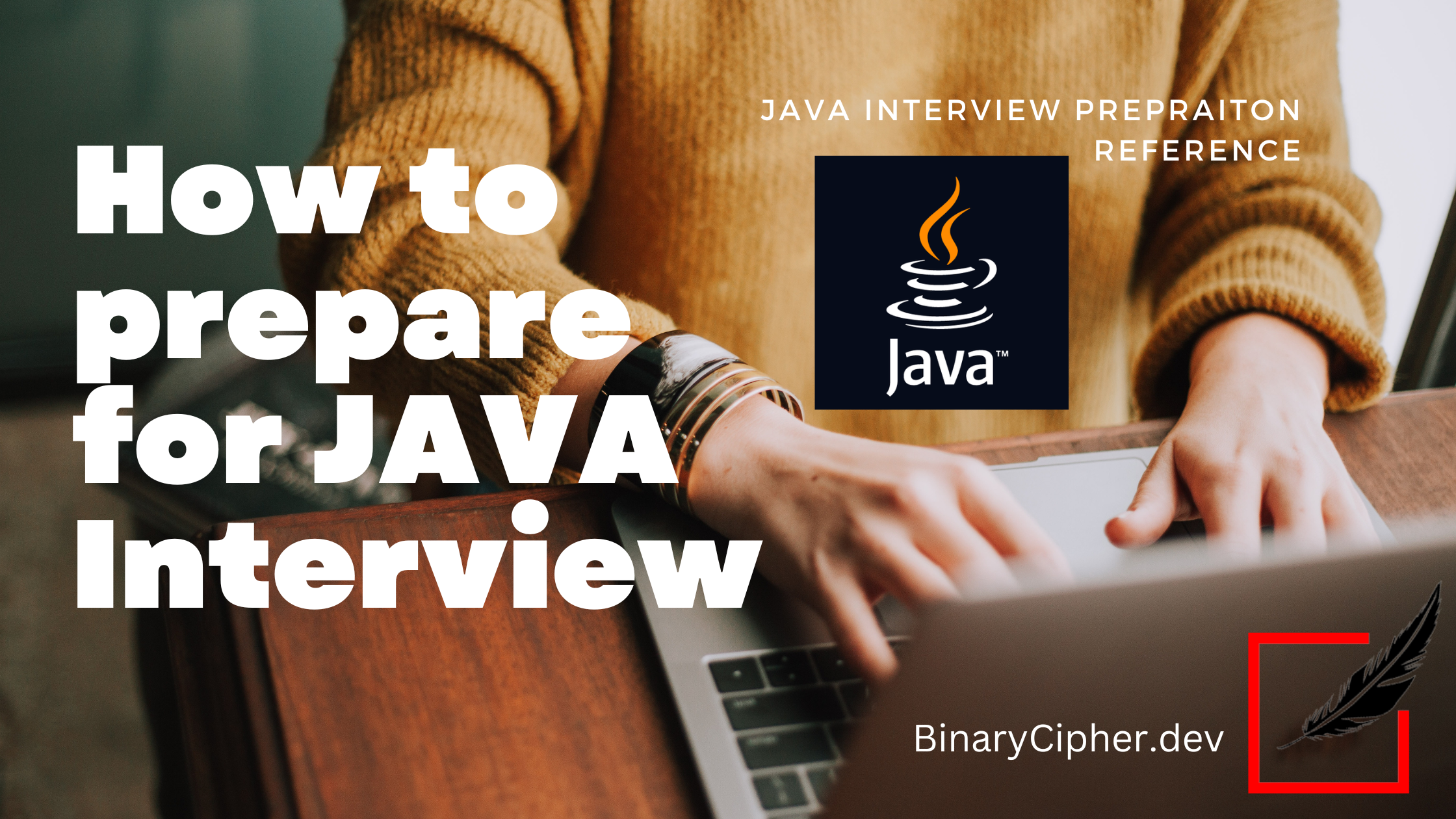
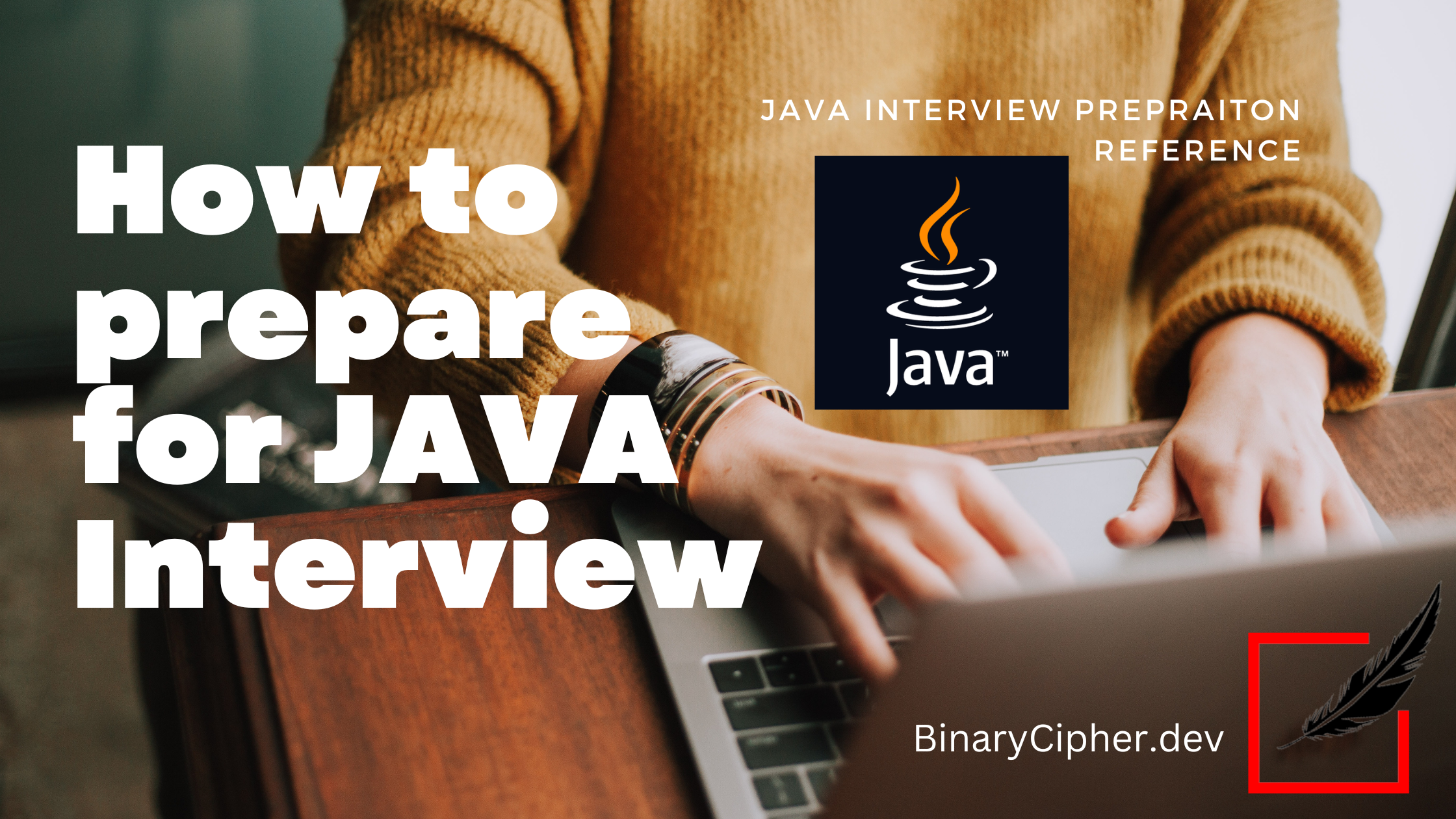
To prepare for a Java interview, you should first review the core concepts and principles of the Java programming language, including its syntax, data types, control structures, object-oriented features, and standard libraries. You should also have a good understanding of common algorithms and data structures, and be able to implement them in Java.
Additionally, you should have a good understanding of software development principles, such as coding best practices, testing, debugging, and problem-solving. You should also be familiar with common Java frameworks and libraries, such as Spring and Hibernate, and be able to discuss their use and benefits.
To prepare for the interview, you can review online tutorials and courses, read books and articles on Java programming, and practice coding problems and challenges. You can also seek advice and guidance from experienced Java developers, and participate in online forums and communities to learn from others and share your own knowledge and experiences.
During the interview, you should be prepared to answer questions about your Java skills and experience, as well as to discuss your previous projects and contributions. You should also be prepared to discuss your problem-solving approach and to write and debug code in a live coding environment. To succeed in the interview, you should be confident, articulate, and focused, and be able to demonstrate your technical skills and expertise in Java programming.
There are many possible Java interview questions, and the specific questions that you will be asked will depend on the specific role and company that you are interviewing for. Here are some common Java interview questions and possible answers:
- What is the Java programming language?
Java is a popular, object-oriented programming language that is used for developing a wide range of applications, from web and mobile apps to enterprise systems and big data analytics. It is known for its reliability, security, and scalability, and is widely used in many different industries.
- What are the key features of Java?
Java is a high-level, platform-independent, and compiled language that is designed to be simple, efficient, and secure. Some of the key features of Java include its object-oriented design, automatic memory management, garbage collection, exception handling, and support for multithreading.
- What are the data types in Java?
Java has a rich set of data types, including primitive types (such as int, float, and boolean) and reference types (such as String, Object, and Array). Primitive types are the basic data types that are built into the language, while reference types are more complex data structures that are defined by the programmer.
- What is an object in Java?
In Java, an object is a collection of data and behavior (methods) that represent a real-world entity. An object has a state (the data it holds) and behavior (the actions it can perform), and it is created from a class, which is a blueprint or template that defines the structure of the object.
- What is inheritance in Java?
Inheritance is a fundamental concept in object-oriented programming that allows one class to inherit the properties and behavior of another class. In Java, inheritance allows a class to extend the functionality of another class, and to reuse and modify its code. This allows for code reuse, modularity, and polymorphism.
- What is polymorphism in Java?
Polymorphism is a concept in object-oriented programming that allows a single interface to be used for different types of objects. In Java, polymorphism is achieved through inheritance, interfaces, and overloading and overriding methods. This allows objects of different types to be treated uniformly, and to have different behavior at runtime.
Here are some sample Java programming questions and answers:
- What is the output of the following Java code?
Copy codeint i = 0;
int j = 5;
while (++i < --j) {
System.out.println(i + " " + j);
}
System.out.println(i + " " + j);
The output of this code is:
Copy code1 4
2 3
3 2
3 2
The while
loop is executed as long as the condition ++i < --j
is true. In each iteration, the values of i
and j
are incremented and decremented, respectively, and then printed. The loop terminates when the condition becomes false, which happens when i
becomes equal to j
. After the loop, the final values of i
and j
are printed.
- What is the output of the following Java code?
Copy codeint[] a = {1, 2, 3};
int[] b = a;
for (int i = 0; i < a.length; i++) {
a[i]++;
}
for (int i = 0; i < b.length; i++) {
System.out.print(b[i] + " ");
}
The output of this code is:
Copy code2 3 4
In this code, the array a
is initialized with the values 1, 2, 3
. The array b
is then assigned the reference of a
, so b
points to the same array as a
. In the first for
loop, the elements of a
are incremented by one. In the second for
loop, the elements of b
are printed. Since b
points to the same array as `a
Here are some of the reference links:
Here is a list of websites that you can use to prepare for a Java interview:
- Java SE API Documentation – https://docs.oracle.com/en/java/javase/
This is the official API documentation for the Java SE platform, and it provides a comprehensive reference for all the classes, interfaces, and methods in the Java language and its standard libraries.
- Java Code Examples – https://www.javacodeexamples.com/
This website provides a large collection of Java code examples and snippets, organized by topic and category. You can browse, search, and learn from these examples to improve your Java skills.
- Java Interview Questions and Answers – https://www.java67.com/2015/07/top-40-java-interview-questions-answers.html
This website provides a list of top 40 Java interview questions and answers, covering a wide range of topics and concepts in Java programming. You can use these questions to test and improve your knowledge and skills.
- Java Interview Prep – https://www.educative.io/collection/page/5668639101419520/5649050225344512/5668600916475904
This website provides a comprehensive course on Java interview preparation, with lessons, quizzes, and practice problems. You can use this course to learn and practice the skills and knowledge that are commonly tested in Java interviews.
- Java Interview Questions – https://www.javaworld.com/article/2076417/java-fundamentals/java-fundamentals-java-101-interview-questions-part-1.html
This website provides a series of articles with Java interview questions and answers, covering various topics in Java
6. LeetCode https://leetcode.com/
7. Geeks for Geeks https://www.geeksforgeeks.org/java/?ref=shm