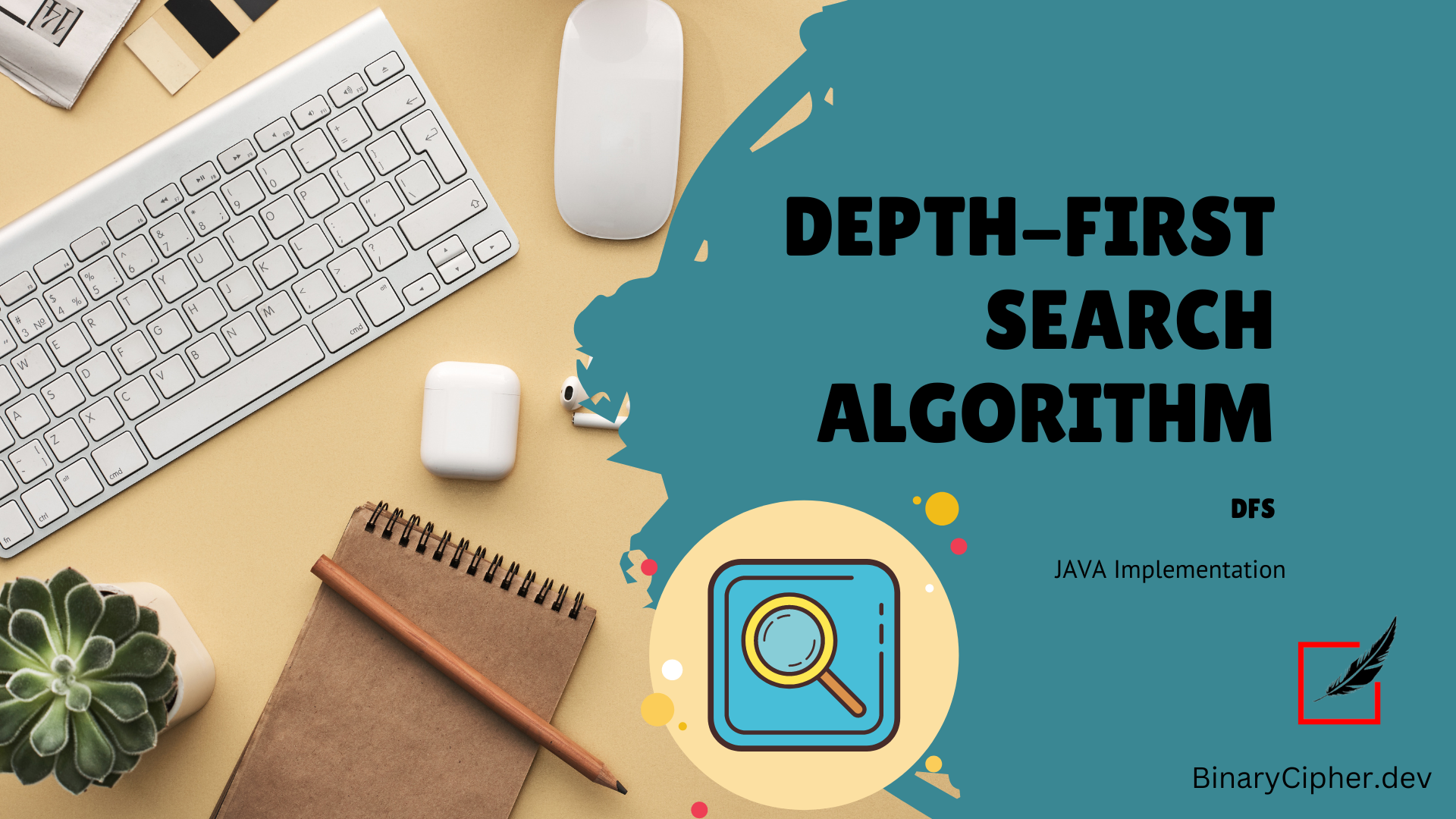
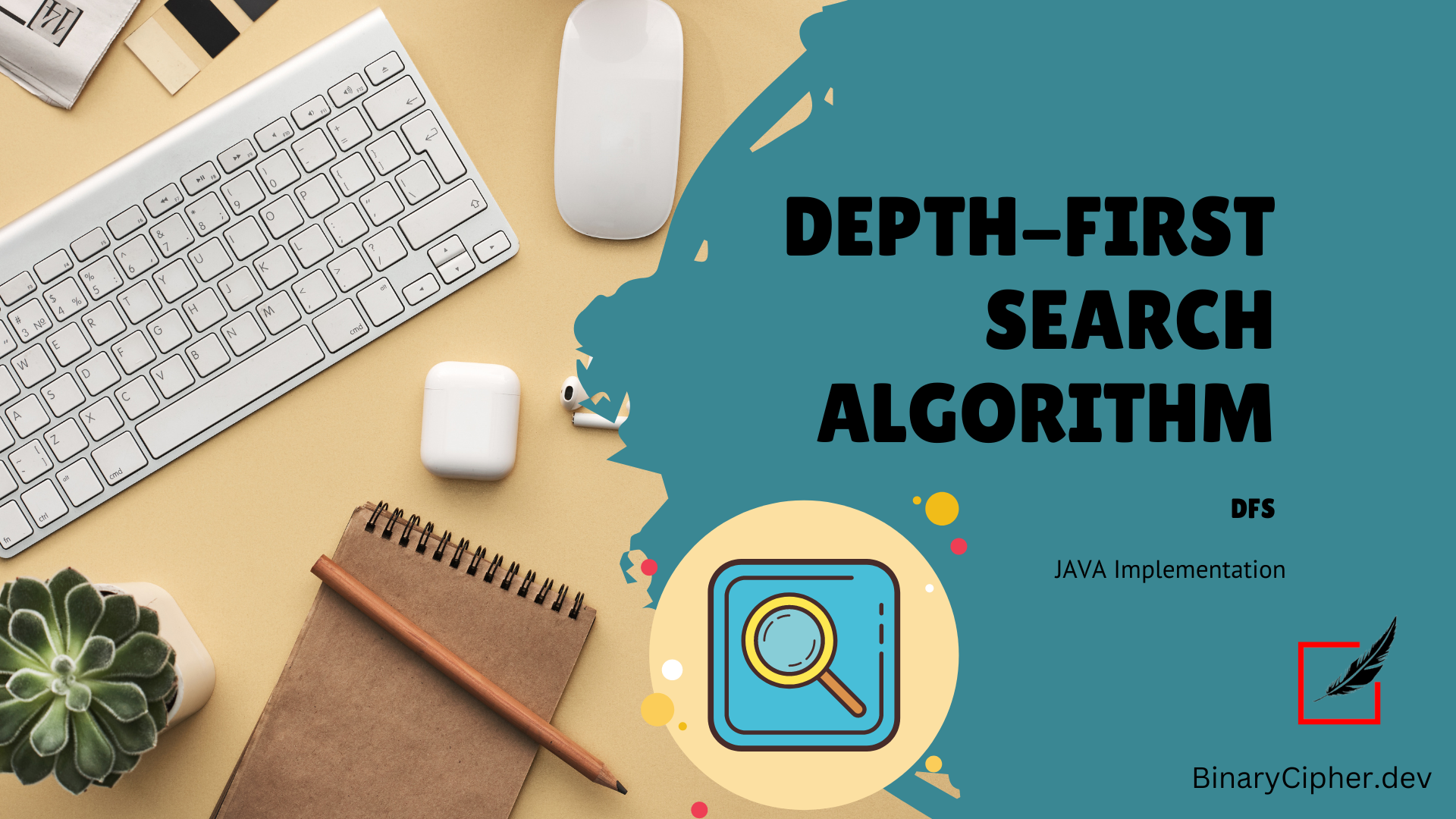
Depth-first search (DFS) is a search algorithm that explores the search tree by going as deep as possible along each branch before backtracking. This means that it explores each branch of the search tree as far as possible before moving on to the next branch.
DFS is useful for many different types of problems, including finding the connected components of a graph, finding paths in a graph, and solving puzzles like mazes and Sudoku.
One of the key advantages of DFS is that it is simple to implement and can be used for many different types of problems. It is also very efficient for small to medium-sized graphs, because it does not explore the entire search space.
However, DFS has some limitations. It can be slow for large graphs, because it may explore a large part of the search space without finding a solution. It can also require a lot of memory, because it stores the entire search path in memory. This can make it difficult to use for very large graphs.
Overall, DFS is a simple and effective search algorithm that is useful for many different types of problems. It is often used in combination with other algorithms to solve more complex problems.
Here is an implementation of the depth-first search (DFS) algorithm in Java:
public class DFS {
public static void search(Node root) {
if (root == null) return;
root.visited = true;
System.out.println(root.value);
for (Node neighbor : root.neighbors) {
if (!neighbor.visited) {
search(neighbor);
}
}
}
}
class Node {
public boolean visited;
public int value;
public Node[] neighbors;
}
In this implementation, the search
method takes a Node
object representing the root of the search tree. It then checks if the root is null and returns if it is. It then marks the root node as visited and prints its value. It then recursively calls the search
method on each of the root’s unvisited neighbors. This continues until all of the nodes in the search tree have been visited.
Differences b/w BFS & DFS
Breadth-first search (BFS) and depth-first search (DFS) are two algorithms used for searching trees, graphs, and other data structures. BFS and DFS are similar in many ways, but there are some key differences between the two algorithms.
One of the main differences between BFS and DFS is the order in which they explore the search space. BFS explores the search space level by level, starting from the root node and moving outward. This means that it visits all of the nodes at the same depth before moving on to the next level. DFS, on the other hand, explores the search space by going as deep as possible along each branch before backtracking. This means that it explores each branch of the search tree as far as possible before moving on to the next branch.
Another key difference between BFS and DFS is their time and space complexity. BFS has a time and space complexity of O(V + E), where V is the number of vertices and E is the number of edges in the graph. This means that the time and space complexity of BFS grows linearly with the size of the input. DFS has a time and space complexity of O(V^2), where V is the number of vertices in the graph. This means that the time and space complexity of DFS grows quadratically with the size of the input.
Overall, BFS and DFS are similar algorithms, but they have some key differences. BFS is more suitable for finding the shortest path between two nodes, while DFS is more suitable for solving puzzles and other problems where the solution is not necessarily the shortest path.
Complexity:
The complexity of an algorithm refers to the amount of resources (such as time and space) that the algorithm uses as the input size grows.
The complexity of the depth-first search (DFS) algorithm is typically O(V^2), where V is the number of vertices in the graph. This means that the time and space complexity of DFS grows quadratically with the size of the input.
The performance of DFS is generally good for small to medium-sized graphs, but it can become slow for very large graphs because it may explore a large part of the search space without finding a solution. It can also require a lot of memory, which can make it difficult to use for very large graphs.
Overall, the complexity and performance of DFS are generally good for small to medium-sized graphs, but it can become slow and require a lot of memory for very large graphs.
Artificial Intelligence: a Revolution in Technology
very good
very good
thanks, interesting read