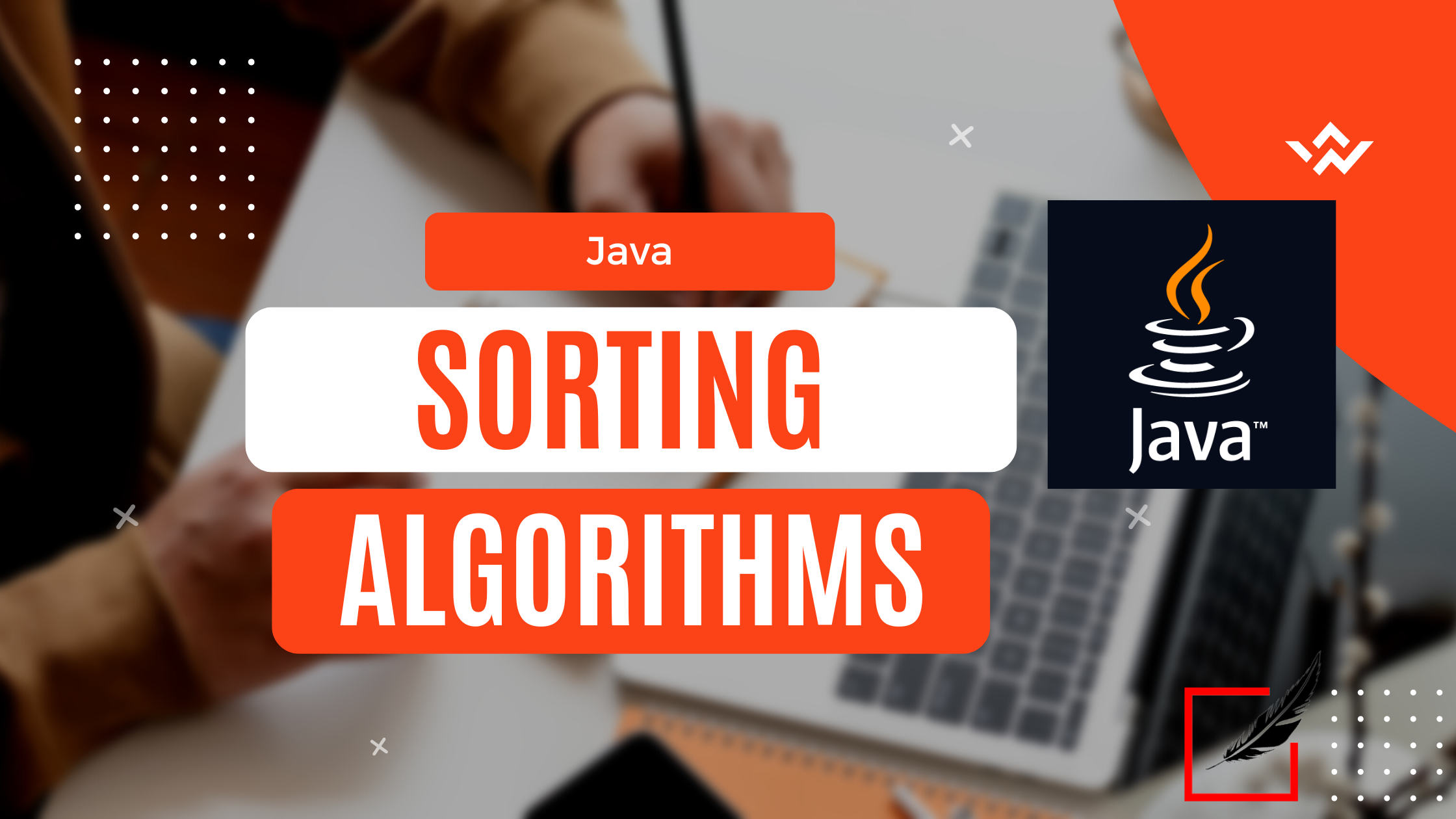
Sorting algorithms are a group of algorithms that are used to rearrange a given set of data in a specific order. There are many different sorting algorithms, each with its own strengths and weaknesses. Some of the most commonly used sorting algorithms include:
- Bubble sort: This is a simple sorting algorithm that repeatedly compares adjacent elements and swaps them if they are in the wrong order.
- Insertion sort: This sorting algorithm sorts the elements by dividing the list into two parts: a sorted part and an unsorted part. The algorithm then repeatedly takes the next element from the unsorted part and inserts it into the correct position in the sorted part.
- Selection sort: This sorting algorithm sorts the elements by repeatedly selecting the smallest element from the unsorted part of the list and adding it to the end of the sorted part.
- Merge sort: This is a divide-and-conquer sorting algorithm that sorts the elements by dividing the list into two smaller lists, sorting each of those lists, and then merging the two sorted lists into one.
- Quick sort: This is another divide-and-conquer sorting algorithm that sorts the elements by selecting a pivot element and partitioning the list into two parts: one with elements that are less than the pivot and one with elements that are greater than the pivot. These two parts are then sorted recursively.
The efficiency of a sorting algorithm is typically measured by the number of comparisons and/or swaps it performs in order to sort a given list. Different sorting algorithms have different time and space complexity, which means that they may be more or less efficient depending on the specific input data.
Bubble sort:
Bubble sort is a simple sorting algorithm that repeatedly compares adjacent elements and swaps them if they are in the wrong order. This process continues until the list is fully sorted.
To understand how bubble sort works, let’s consider an example. Suppose we have the following list of numbers that we want to sort in ascending order:
[5, 2, 4, 1, 3]
We can use bubble sort to sort this list as follows:
- Compare the first two elements (5 and 2) and swap them if they are in the wrong order. In this case, 5 is greater than 2, so we need to swap them. The list now becomes [2, 5, 4, 1, 3].
- Compare the second and third elements (5 and 4) and swap them if they are in the wrong order. In this case, 5 is greater than 4, so we need to swap them. The list now becomes [2, 4, 5, 1, 3].
- Compare the third and fourth elements (5 and 1) and swap them if they are in the wrong order. In this case, 5 is greater than 1, so we need to swap them. The list now becomes [2, 4, 1, 5, 3].
- Compare the fourth and fifth elements (5 and 3) and swap them if they are in the wrong order. In this case, 5 is greater than 3, so we need to swap them. The list now becomes [2, 4, 1, 3, 5].
- Since we have reached the end of the list, we need to go back to the beginning and repeat the process. We start again by comparing the first two elements (2 and 4) and swapping them if they are in the wrong order. In this case, there is no need to swap them since they are already in the correct order.
- Compare the second and third elements (4 and 1) and swap them if they are in the wrong order. In this case, 4 is greater than 1, so we need to swap them. The list now becomes [2, 1, 4, 3, 5].
- Compare the third and fourth elements (4 and 3) and swap them if they are in the wrong order. In this case, 4 is greater than 3, so we need to swap them. The list now becomes [2, 1, 3, 4, 5].
- Compare the fourth and fifth elements (4 and 5) and swap them if they are in the wrong order. In this case, there is no need to swap them since they are already in the correct order.
- Since we have reached the end of the list again, we need to go back to the beginning and repeat the process once more. We start again by comparing the first two elements (2 and 1) and swapping them if they are in the wrong order. In this case, 2 is greater than 1, so we need to swap them. The list now becomes [1, 2, 3, 4, 5].
- Compare the second and third elements (2 and 3) and swap them if they are in the wrong order. In this case, there is no need to swap them since they are already in the correct order.
- Compare the third and fourth elements (3 and 4) and swap them if they are in the wrong order. In this case, there is no need to swap them since they are already in the correct order.
- Compare the fourth and fifth elements (4 and 5) and swap them if they are in the wrong order. In this case
public class BubbleSort {
public static void sort(int[] arr) {
// Flag to keep track of whether any swaps have occurred
boolean swapped = true;
// Keep looping until no swaps have occurred
while (swapped) {
// Set the flag to false
swapped = false;
// Loop through the array and compare adjacent elements
for (int i = 0; i < arr.length - 1; i++) {
// If the current element is greater than the next element, swap them
if (arr[i] > arr[i + 1]) {
int temp = arr[i];
arr[i] = arr[i + 1];
arr[i + 1] = temp;
// Set the flag to true since a swap has occurred
swapped = true;
}
}
}
}
public static void main(String[] args) {
// Test the bubble sort algorithm
int[] arr = {5, 2, 4, 1, 3};
sort(arr);
System.out.println(Arrays.toString(arr)); // Output: [1, 2, 3, 4, 5]
}
}
In this program, the sort()
method takes an array of integers as input and uses the bubble sort algorithm to sort the array in ascending order. The method uses a flag variable to keep track of whether any swaps have occurred. If no swaps have occurred, the list is already sorted, and the method can return. Otherwise, the method repeats the process of comparing adjacent elements and swapping them if they are in the wrong order. This process continues until no more swaps are needed, at which point the list is fully sorted.
The main()
method of the program tests the sort()
method by calling it with an array of unsorted numbers. After the sort()
method has been called, the main()
method prints the sorted array to the console.
Insertion sort:
Insertion sort is a simple sorting algorithm that sorts the elements by dividing the list into two parts: a sorted part and an unsorted part. The algorithm then repeatedly takes the next element from the unsorted part and inserts it into the correct position in the sorted part.
To understand how insertion sort works, let’s consider an example. Suppose we have the following list of numbers that we want to sort in ascending order:
[5, 2, 4, 1, 3]
We can use insertion sort to sort this list as follows:
- Start by considering the first element in the list (5) as the sorted part, and the remaining elements (2, 4, 1, 3) as the unsorted part.
- Take the next element from the unsorted part (2) and insert it into the correct position in the sorted part. Since 2 is less than 5, we need to insert it at the beginning of the sorted part. The list now becomes [2, 5, 4, 1, 3].
- Take the next element from the unsorted part (4) and insert it into the correct position in the sorted part. Since 4 is greater than 2 but less than 5, we need to insert it between those two elements. The list now becomes [2, 4, 5, 1, 3].
- Take the next element from the unsorted part (1) and insert it into the correct position in the sorted part. Since 1 is less than 2, we need to insert it at the beginning of the sorted part. The list now becomes [1, 2, 4, 5, 3].
- Take the next element from the unsorted part (3) and insert it into the correct position in the sorted part. Since 3 is greater than 1 but less than 2, we need to insert it between those two elements. The list now becomes [1, 2, 3, 4, 5].
At this point, the entire list is considered to be sorted, so the algorithm can stop. The final sorted list is [1, 2, 3, 4, 5].
Insertion sort is a simple and intuitive algorithm, and it can be easily implemented in any programming language. It has a time complexity of O(n^2), which means that it is not very efficient for sorting large lists. However, it has the advantage of being a stable sorting algorithm, which means that it preserves the relative order of elements with equal values. This can be useful in some cases.
public class InsertionSort {
public static void sort(int[] arr) {
// Loop through the array and insert each element into the correct position in the sorted part
for (int i = 0; i < arr.length; i++) {
int current = arr[i];
int j = i - 1;
// Find the correct position for the current element in the sorted part
while (j >= 0 && arr[j] > current) {
arr[j + 1] = arr[j];
j--;
}
// Insert the current element into the correct position
arr[j + 1] = current;
}
}
public static void main(String[] args) {
// Test the insertion sort algorithm
int[] arr = {5, 2, 4, 1, 3};
sort(arr);
System.out.println(Arrays.toString(arr)); // Output: [1, 2, 3, 4, 5]
}
}
In this program, the sort()
method takes an array of integers as input and uses the insertion sort algorithm to sort the array in ascending order. The method loops through the array and takes each element in turn. For each element, it finds the correct position for that element in the sorted part of the array, and inserts it into that position.
The main()
method of the program tests the sort()
method by calling it with an array of unsorted numbers. After the sort()
method has been called, the main()
method prints the sorted array to the console.
Selection Sort:
Selection sort is a simple sorting algorithm that sorts the elements by repeatedly selecting the smallest element from the unsorted part of the list and adding it to the end of the sorted part.
To understand how selection sort works, let’s consider an example. Suppose we have the following list of numbers that we want to sort in ascending order:
[5, 2, 4, 1, 3]
We can use selection sort to sort this list as follows:
- Start by considering the entire list as the unsorted part, and an empty list as the sorted part.
- Find the smallest element in the unsorted part of the list (1) and add it to the end of the sorted part. The sorted part now becomes [1] and the unsorted part becomes [5, 2, 4, 3].
- Find the smallest element in the unsorted part of the list (2) and add it to the end of the sorted part. The sorted part now becomes [1, 2] and the unsorted part becomes [5, 4, 3].
- Find the smallest element in the unsorted part of the list (3) and add it to the end of the sorted part. The sorted part now becomes [1, 2, 3] and the unsorted part becomes [5, 4].
- Find the smallest element in the unsorted part of the list (4) and add it to the end of the sorted part. The sorted part now becomes [1, 2, 3, 4] and the unsorted part becomes [5].
- Find the smallest element in the unsorted part of the list (5) and add it to the end of the sorted part. The sorted part now becomes [1, 2, 3, 4, 5] and the unsorted part becomes empty.
At this point, the entire list is considered to be sorted, so the algorithm can stop. The final sorted list is [1, 2, 3, 4, 5].
public class SelectionSort {
public static void sort(int[] arr) {
// Loop through the array and find the smallest element in each iteration
for (int i = 0; i < arr.length - 1; i++) {
int minIndex = i;
// Find the index of the smallest element in the unsorted part of the array
for (int j = i + 1; j < arr.length; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
// Swap the smallest element with the first element in the unsorted part of the array
int temp = arr[i];
arr[i] = arr[minIndex];
arr[minIndex] = temp;
}
}
public static void main(String[] args) {
// Test the selection sort algorithm
int[] arr = {5, 2, 4, 1, 3};
sort(arr);
System.out.println(Arrays.toString(arr)); // Output: [1, 2, 3, 4, 5]
}
}
In this program, the sort()
method takes an array of integers as input and uses the selection sort algorithm to sort the array in ascending order. The method loops through the array and finds the smallest element in the unsorted part of the array. It then swaps that element with the first element in the unsorted part of the array. This process continues until the entire array is sorted.
The main()
method of the program tests the sort()
method by calling it with an array of unsorted numbers. After the sort()
method has been called, the main()
method prints the sorted array to the console.
Merge sort:
Merge sort is a divide-and-conquer sorting algorithm that sorts the elements by dividing the list into two smaller lists, sorting each of those lists, and then merging the two sorted lists into one.
To understand how merge sort works, let’s consider an example. Suppose we have the following list of numbers that we want to sort in ascending order:
[5, 2, 4, 1, 3]
We can use merge sort to sort this list as follows:
- Divide the list into two smaller lists by splitting it in the middle. The two lists are [5, 2, 4] and [1, 3].
- Sort each of the two smaller lists by applying the merge sort algorithm recursively. The two sorted lists are [2, 4, 5] and [1, 3].
- Merge the two sorted lists into one by comparing the first element of each list and adding the smaller element to the result list. In this case, the smaller element is 1, so we add it to the result list. The result list now becomes [1] and the two lists to be merged become [2, 4, 5] and [3].
- Repeat step 3 until all elements from both lists have been added to the result list. The result list now becomes [1, 2, 3, 4, 5].
At this point, the entire list is considered to be sorted, so the algorithm can stop. The final sorted list is [1, 2, 3, 4, 5].
Merge sort is a highly efficient sorting algorithm with a time complexity of O(n log n). It is also a stable sorting algorithm, which means that it preserves the relative order of elements with equal values. This can be useful in some cases. However, the algorithm requires additional space to store the two smaller lists while they are being sorted, which can be a disadvantage in some situations.
public class MergeSort {
public static void sort(int[] arr) {
// Check if the array has more than one element
if (arr.length > 1) {
// Divide the array in half
int mid = arr.length / 2;
int[] left = Arrays.copyOfRange(arr, 0, mid);
int[] right = Arrays.copyOfRange(arr, mid, arr.length);
// Sort the two halves of the array
sort(left);
sort(right);
// Merge the two sorted halves of the array
int i = 0;
int j = 0;
int k = 0;
while (i < left.length && j < right.length) {
if (left[i] < right[j]) {
arr[k] = left[i];
i++;
} else {
arr[k] = right[j];
j++;
}
k++;
}
// Add any remaining elements from the left half of the array
while (i < left.length) {
arr[k] = left[i];
i++;
k++;
}
// Add any remaining elements from the right half of the array
while (j < right.length) {
arr[k] = right[j];
j++;
k++;
}
}
}
public static void main(String[] args) {
// Test the merge sort algorithm
int[] arr = {5, 2, 4, 1, 3};
sort(arr);
System.out.println(Arrays.toString(arr)); // Output: [1, 2, 3, 4, 5]
}
}
In this program, the sort()
method takes an array of integers as input and uses the merge sort algorithm to sort the array in ascending order.
Quick sort:
Quick sort is a divide-and-conquer sorting algorithm that sorts the elements by selecting a pivot element from the list and partitioning the list into two smaller lists: one containing the elements that are smaller than the pivot, and the other containing the elements that are greater than or equal to the pivot. The algorithm then applies itself recursively to each of the two smaller lists to sort them.
To understand how quick sort works, let’s consider an example. Suppose we have the following list of numbers that we want to sort in ascending order:
[5, 2, 4, 1, 3]
We can use quick sort to sort this list as follows:
- Choose a pivot element from the list. In this case, we will choose the first element (5).
- Partition the list into two smaller lists by comparing each element to the pivot. The elements that are smaller than the pivot are placed in one list, and the elements that are greater than or equal to the pivot are placed in the other list. In this case, the two lists are [2, 4, 1, 3] and [5].
- Apply the quick sort algorithm recursively to each of the two smaller lists to sort them. For the first list, we choose the first element (2) as the pivot and partition the list into two smaller lists: [1] and [4, 3]. We then apply the quick sort algorithm to each of these smaller lists. For the second list, we choose the first element (5) as the pivot and partition the list into two smaller lists: [] and [5]. We then apply the quick sort algorithm to the second list.
- Merge the two sorted lists into one. In this case, the two lists are [1, 2, 3, 4] and [5]. We can merge these two lists by simply concatenating them, resulting in the final sorted list [1, 2, 3, 4, 5].
Quick sort is a highly efficient sorting algorithm with a time complexity of O(n log n) on average. It has the advantage of being an in-place sorting algorithm, which means that it does not require additional space to sort the elements. However, the algorithm can be less efficient in the worst case, with a time complexity of O(n^2). This can happen if the pivot element is chosen poorly, for example if it is the largest or smallest element in the list. To avoid this, it is important to choose the pivot element carefully.
public class QuickSort {
public static void sort(int[] arr, int left, int right) {
// Check if the list has more than one element
if (left < right) {
// Choose the pivot element and partition the list into two smaller lists
int pivotIndex = partition(arr, left, right);
// Sort the left and right halves of the list recursively
sort(arr, left, pivotIndex - 1);
sort(arr, pivotIndex + 1, right);
}
}
private static int partition(int[] arr, int left, int right) {
// Choose the pivot element as the middle element in the list
int pivot = arr[(left + right) / 2];
// Move the pivot element to the end of the list
int temp = arr[right];
arr[right] = arr[pivot];
arr[pivot] = temp;
// Partition the list into two smaller lists
int i = left;
int j = right - 1;
while (i < j) {
// If the current element is smaller than the pivot, move it to the left list
if (arr[i] < pivot) {
i++;
} else {
// If the current element is greater than or equal to the pivot, move it to the right list
temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
j--;
}
}
// Move the pivot element back to the middle of the list
temp = arr[i];
arr[i] = arr[right];
arr[right] = temp;
// Return the index of the pivot element
return i;
}
public static void main(String[] args) {
// Test the quick sort algorithm
int[] arr = {5, 2, 4, 1, 3};
sort(arr, 0, arr.length - 1);
System.out.println(Arrays.toString(arr)); // Output: [1, 2, 3, 4, 5]
}
}
In this program, the sort()
method takes an array of integers as input and uses the quick sort algorithm to sort the array in ascending order. The method first checks if the array has more than one element. If it does, it partitions the array into two smaller lists using the partition()
method and applies itself recursively to each of the two smaller lists to sort them.
The partition()
method takes the array, the left and right indices of the list to be partitioned, and returns the index of the pivot element. It first chooses the pivot element as the middle element in the list and moves it to the end of the list. It then partitions the list into two smaller lists by
Compare all sorting Algorithms:
There are several different sorting algorithms, each with its own strengths and weaknesses. Here is a comparison of some of the most common sorting algorithms:
- Bubble sort is a simple sorting algorithm that sorts the elements by repeatedly comparing adjacent elements and swapping them if they are in the wrong order. It has a time complexity of O(n^2) and is not very efficient for large lists. However, it is easy to understand and implement.
- Insertion sort is a simple sorting algorithm that sorts the elements by inserting each element into the correct position in the sorted part of the list. It has a time complexity of O(n^2) and is not very efficient for large lists. However, it is efficient for small lists and for lists that are almost sorted.
- Selection sort is a simple sorting algorithm that sorts the elements by repeatedly selecting the smallest element from the unsorted part of the list and adding it to the end of the sorted part. It has a time complexity of O(n^2) and is not very efficient for large lists. However, it is efficient for small lists and for lists that are almost sorted.
- Merge sort is a divide-and-conquer sorting algorithm that sorts the elements by dividing the list into two smaller lists, sorting each of those lists, and then merging the two sorted lists into one. It has a time complexity of O(n log n) and is highly efficient for large lists. However, it requires additional space to store the two smaller lists while they are being sorted.
- Quick sort is a divide-and-conquer sorting algorithm that sorts the elements by selecting a pivot element from the list and partitioning the list into two smaller lists: one containing the elements that are smaller than the pivot, and the other containing the elements that are greater than or equal to the pivot. It has a time complexity of O(n log n) on average and is highly efficient for large lists. However, it can be less efficient in the worst case, with a time complexity of O(n^2). It also requires additional space to store the two smaller lists while they are being sorted.
In general, merge sort and quick sort are the most efficient sorting algorithms for large lists, while bubble sort, insertion sort, and selection sort are more efficient for small lists and for lists that are almost sorted. The choice of sorting algorithm depends on the specific requirements of the task at hand.